목록알고리즘 (266)
Dev.baelanche
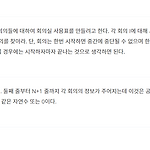
그리디 알고리즘 문제로 정렬 기준을 잘 잡아야 한다. 회의 정보에 대하여 1. 회의가 끝나는 시간이 가장 빠른순으로 오름차순 정렬한다. 2. 회의가 끝나는 시간이 같은 회의가 있다면 회의 시작시간이 빠른 것을 앞으로 한다. 많은 정렬 방법이 있겠지만 Comparator 를 구현하여 정렬했다. public class Main { public static void main(String[] args) { Scanner sc = new Scanner(System.in); int n = sc.nextInt(); int a[][] = new int[n][2]; for(int i=0; i
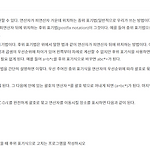
다음과 같은 규칙을 따라 스택으로 구현해야 한다. 1. A, B, C .... 등의 피연산자는 곧바로 출력한다. 2. +, - 연산자는 push 한다. - 그렇지 않을때는 위 조건이 참이 될때까지 스택을 pop 하며 출력한다. 3. *, / 연산자는 push 한다. - 그렇지 않을때는 위 조건이 참이 될때까지 스택을 pop 하며 출력한다. 4. ( 기호는 push 한다. 5. ) 기호는 스택의 탑이 '(' 이 될때까지 스택을 pop 하며 출력한다. - '(' 기호는 pop 하지만 출력하지는 않는다. 6. 위 절차가 끝나고 스택에 값이 남아있다면 모두 pop 하며 출력한다. public class Main { public static void main(String[] args) { Scanner sc = ..
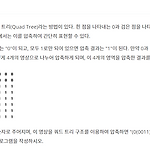
1780번 문제와 동일한 방식으로 풀면된다. 다만 재귀가 깊어질수록 출력할 숫자 좌우에 괄호를 붙여주어야 하는데, 필자는 큐에 담아서 pop 하는 방식으로 구현했다. public class Main { static char[][] a; static Queue q = new LinkedList(); public static void main(String[] args) { Scanner sc = new Scanner(System.in); int n = sc.nextInt(); a = new char[n][n]; for(int i=0; i
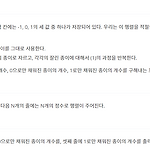
분할정복을 사용하는 전형적인 문제이다. 종이의 크기를 9로 예를 들면 1. 9*9 를 탐색한다. 2. 종이가 모두 같은 수로 되어있지 않다면 9등분하여 각 종이를 탐색한다. 3. 2번을 반복하여 -1, 0, 1 의 개수를 센다. 2번이 계속 반복되므로 재귀함수로 짜주기만 하면 된다. 코드를 보고 머리로 그려보는 것이 좋을 것 같다. public class Main { static int a[][]; static int cnt[] = new int[3]; public static void main(String[] args) { Scanner sc = new Scanner(System.in); int n = sc.nextInt(); a = new int[n][n]; for(int i=0; i
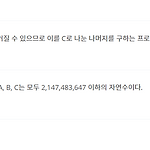
x제곱 하기에 수가 너무 크므로 분할하는 방법으로 접근해야 한다. B를 2로 계속 나누어 최대한 작은 수로 만들고 매 연산마다 (A*B)%C = ((A%C)*(B%C))%C 를 이용하여 수를 더 작게 만들어준다. public class Main { public static void main(String[] args) { Scanner sc = new Scanner(System.in); int a = sc.nextInt(); int b = sc.nextInt(); int c = sc.nextInt(); long ans = 1; long mul = a % c; while(b>0) { if(b%2 == 1) { ans *= mul; ans %= c; } mul = ((mul % c) * (mul % c)) %..
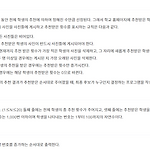
규칙에 따라 구현하는 문제이다. 사진틀 정보를 담을 배열은 순서가 보장되고 동적크기를 가진 ArrayList 를 사용했다. 학생 번호와 추천수를 담은 객체를 사용하였고 번호에 따른 정렬을 내부에서 구현했다. public class Main { public static void main(String[] args) { Scanner sc = new Scanner(System.in); int n = sc.nextInt(); ArrayList list = new ArrayList(); for(int i=0; i0) { int rec = sc.nextInt(); boolean st = false; for(int i=0; i
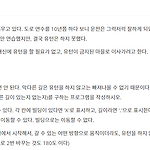
유턴을 하지 않으려면 갈 수 있는 방향이 적어도 2개 이상이어야 한다. 길마다 이동가능한 방향이 2가지 이상인지 확인하면 된다. public class Main { public static void main(String[] args) { Scanner sc = new Scanner(System.in); int r = sc.nextInt(); int c = sc.nextInt(); char a[][] = new char[r][c]; for(int i=0; i
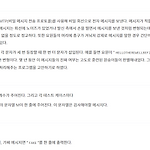
입력된 메세지를 알고리즘대로 체크해 새 메세지를 만들어내고 둘을 비교하는 방식으로 풀었다. public class Main { public static void main(String[] args) { Scanner sc = new Scanner(System.in); int t = sc.nextInt(); while(t-->0) { String str = sc.next(); char c[] = str.toCharArray(); int alpha[] = new int[26]; StringBuilder sb = new StringBuilder(); for(int i=0; i